Industry Standard API Mocking for JavaScript.
Mock Service Worker is an API mocking library that allows you to write client-agnostic mocks and reuse them across any frameworks, tools, and environments.
Making tests better at
Control the network
Focus on describing how the network should behave instead of thinking how to mock your request client.
import { http, HttpResponse } from 'msw'import { setupWorker } from 'msw/browser' // Describe the network.const handlers = [ http.get('https://acme.com/product/:id', ({ params }) => { return HttpResponse.json({ id: params.id, title: 'Porcelain Mug', price: 9.99, }) }),] // Enable API mocking anywhere.const worker = setupWorker(...handlers)await worker.start()
-
Omit implementation details
Intercept both REST and GraphQL API requests regardless of how they were made. Use request clients that suit your product, not your API mocking tool of choice.
-
Use the platform
Handle requests and responses using the standard Fetch API. Respect the web standards and invest your time into learning the platform, not the tools.
-
Reuse like never before
No configurations, adapters, or plugins. Reuse the same mocks across environments and tools, be it an integration test with Vitest, an automated browser test with Playwright, a demo showcase in Storybook, or a React Native app. Or all of them at once.
I found MSW and was thrilled that not only could I still see the mocked responses in my DevTools, but that the mocks didn't have to be written in a Service Worker and could instead live alongside the rest of my app. This made it silly easy to adopt. The fact that I can use it for testing as well makes MSW a huge productivity booster.
Kent C. Dodds
Software Engineer and Educator
Integrate anywhere
A single source of truth for your network across the entire stack.
Porcelain Mug
$9.99
Local development
Create, change, and debug fast by augmenting existing APIs—both third-party and local—or designing them as you go.
$ vitest
❯ components/ProductDetail.test.ts
✓ displays product name
✓ displays net price
✓ handles network errors
Tests 3 passed (3)
Duration 100ms
Integration tests
Test happy paths and override network behavior on a per-test basis to test even the most trickest of edge cases.
Product detail page
✔Displays product item details
Test body
1 Visit /product/abc-123 (XHR) GET 200 api.acme/product/abc-123 2 Assert expected h1.title to have value Porcelain Mug 3 Assert expected p.price to have value $9.99
End-to-end tests
Take any HTTP call out of your test's equation and focus on the user experience, mocking local or external APIs.
- Components
+ProductDetail
- Idle
- Network error
- Added to cart
Storybook showcase
Emulate the precise network state to share how your components handle it with the entire team.
Mock Service Worker has become a fundamental part of my development and testing workflow. With MSW I don't have to worry about endpoints or databases being down or slow. And I can forget about brittle tests due to changing data. I configure mocks that are 100% reliable and predictable. The result? Faster development and rock-solid automated UI tests.
Cory House
Software Architect
Community
Feedback
What developers are saying.
I've spent numerous hours mocking and stubbing data for tests over the years wishing for a better approach. I was recently introduced to MSW and love how it instantly improves the developer experience.
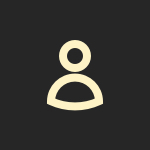
Alffrey Chemmannoor
Software Engineer
We've switched our tests over to use Mock Service Worker and we haven't looked back ever since.
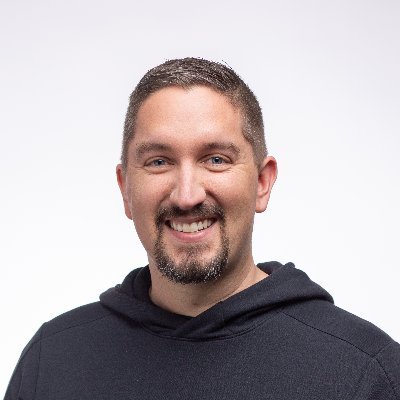
Michael Haglund
Engineering Manager
I don't think I can use anything other than MSW after picking it up for a project at my previous workplace, to be honest.
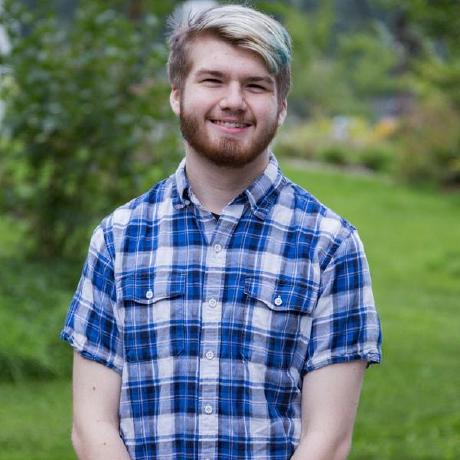
Brady Pascoe
Maintainer of React Bootstrap
Mock Service Worker is the best thing that ever happened to the JS community. Sharing mocks between development, unit, and E2E tests has never been that easy.
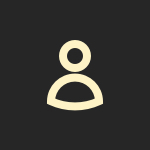
Tobias Pickel
Software Engineer
Just a shoutout to MSW for being an absolute dream to work with. Simple to get running and it's allowed me to continue working while the backend was down. Much faster than writing a mock server.
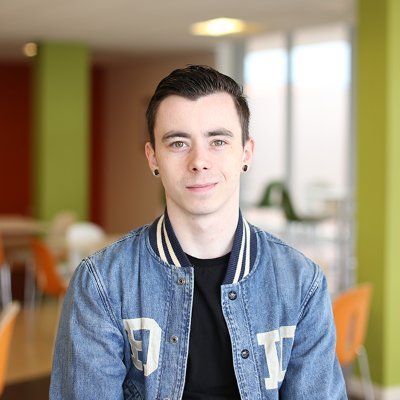
Konna Buraun
Software Engineer
I can't even imagine the mock I'd have to contrive to simulate a server sending a CSRF cookie, and with MSW I don't have to!
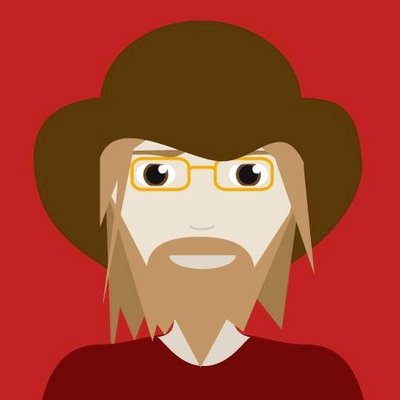
Derek DeHart
Software Engineer
Mock Service Worker was a life-saver for me when we had a strict deadline and there was no backend.
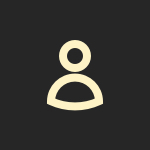
Rafal Rudol
Senior Frontend Developer
It's quite a genius use of Service Workers—works for both REST and GraphQL API.
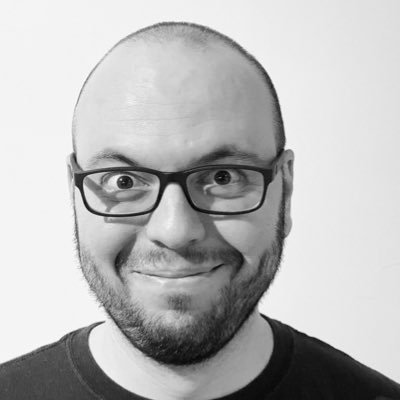
Heitor Lessa
AWS EMEA
When it comes to mocking, I always recommend Mock Service Worker. It's amazing and as close as it gets to plug & play.
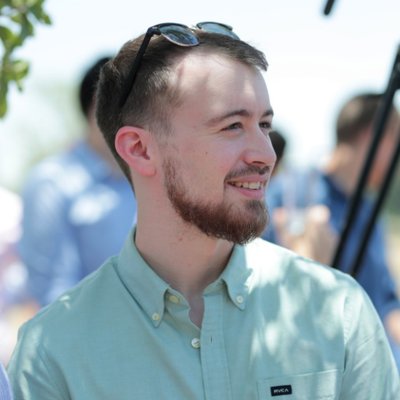
Matan Borenkraout
Frontend Engineer
Ship better products today.
Mock Service Worker is the best way to integrate API mocking across your entire stack. Test, prototype, and debug withouth sacrificing your application's integrity. Give it a try, it's open-source and free!
Get started in 3 steps